How to import a Github Package into Gradle projects
Github packages allows you to generate packages from repositories in a simple and fast way. However, after deploying a package into the Package Registry, include the package inside a Java Gradle project may be a bit tricky.
In this article we will se:
- how to create a Github personal access token
- how to use the access token to import our package into a Java Gradle project
- including Github package into Gradle using
System.getenv
- including Github package into Gradle using properties (best solution)
Let’s see together how!
Create a Github personal access token
First, you need to generate a personal Github access token. To do so, we need to go to our Github account Settings, then, in the left sidebar, go to Developer settings:
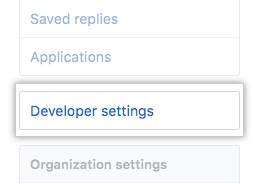
In the left sidebar, go to Personal access tokens:
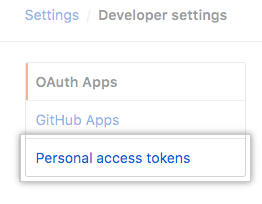
Once we are there, we need to click on Generate new token (prompt your user credential if needed):

Inside the Note field, enter a description to help you remember where you will use that token:
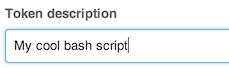
Now select permissions you want to grant to your token. In order to allow us to access to the packages of our repository using the token, we need to give the repo permissions:
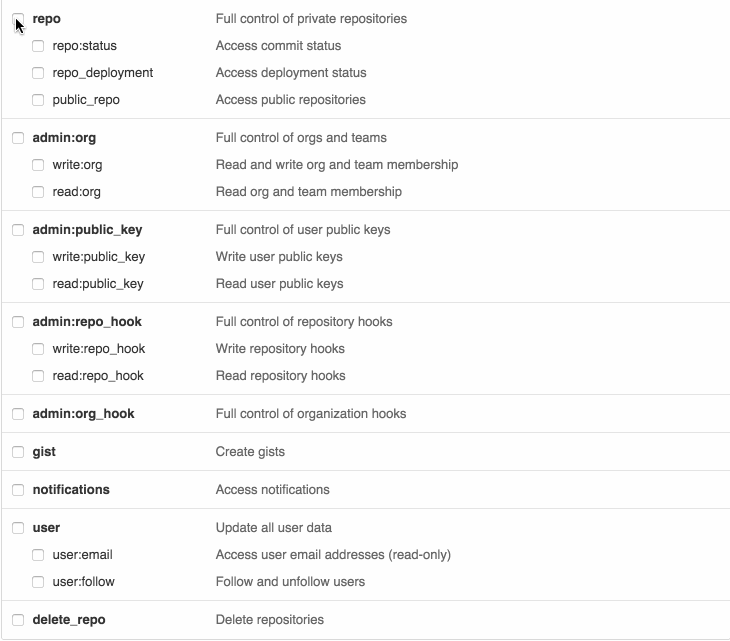
Finally, click Generate token:
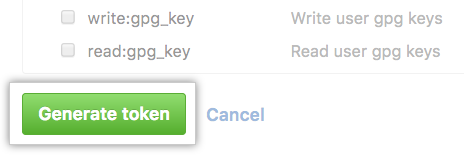
Once we have clicked, we will be shown the token. Important! Be sure to copy it in a safe place because you will not be able to see the token again.
Import Github package into Gradle project
First, we need to go to our repository where we have already published a Java Github package. If we have a package into our repository, we should see something similar on the right sidebar:
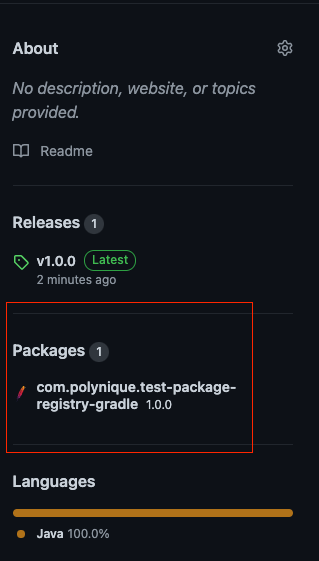
Another way to reach our package is to go to https://github.com/OWNER/REPOSITORY/package
url, that we can click on the Github package that we want to include into our Gradle project. At this point, we should see something like this:
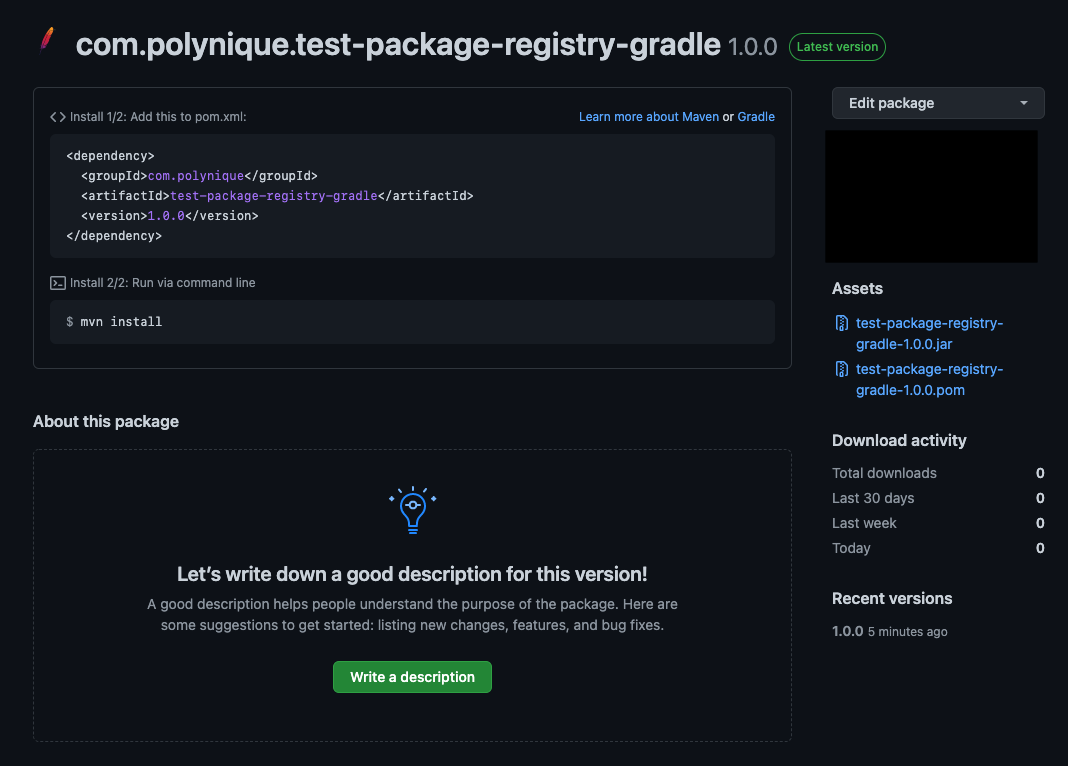
Include package into Gradle project using System.getenv
One easy way to include the package is to insert the following lines into our build.gradle
file:
plugins {
id 'maven'
}
repositories {
maven {
name = "GitHubPackages"
url = uri("https://maven.pkg.github.com/OWNER/REPOSITORY")
credentials {
username = project.findProperty("gpr.user") ?: System.getenv("USERNAME")
password = project.findProperty("gpr.key") ?: System.getenv("TOKEN")
}
}
}
dependencies {
compile 'com.example:package:version'
}
Include package into Gradle project using property file
Including a Github package into a Gradle project using a property file is the method I personally prefer because it allows us to have a little more control.
Firstly, we need to create an .env
file into the root directory or our project. Inside the file, we put our Github username and the token we have previously generated:
USERNAME=your-username
TOKEN=yourtoken
Don’t forget to add the .env
file to your .gitignore
!
Now, we edit our build.gradle
file so that we can import the .env
file we previously added to the project:
plugins {
id 'maven'
}
def props = new Properties()
file(".env").withInputStream { props.load(it) }
repositories {
mavenCentral()
maven {
name = "GitHubPackages"
url = uri("https://maven.pkg.github.com/OWNER/REPOSITORY")
credentials {
username = project.findProperty("gpr.user") ?: props.getProperty("USERNAME")
password = project.findProperty("gpr.key") ?: props.getProperty("TOKEN")
}
}
}
dependencies {
compile 'com.example:package:version'
}
Thank to the few additions we have made, we no longer need to manage environment variables, but we just need to keep our credentials in a properties file that is only in our development environment.
Conclusion
Firstly, we have seen how to create a personal Github access token and how to use it to include a Github package within a Java Gradle project. Then, we have used two different methods to include a package into a Gradle project: using environment variables and using a property file.
External resources: