Java String to Date and Date to String
How to convert a Date to String and parse a Date from a String in Java? We can use the SimpleDateFormat class to convert a Date to String and vice versa. In particular, using the format and parse methods we can, respectively, parse a string and format a date.
In the next chapters, we will see how to use these two methods to:
You can find in this Github repository the full code shown in this article.
What is SimpleDateFormat?
SimpleDateFormat is a Java class that is used to format and parse a Date using a specific date and time pattern. It comes from the java.text.SimpleDateFormat
package and is included by default from Java Platform SE 7. In this guide, we will make extensive use of the “format” and “parse” methods because they are the main methods that allow us to transform Dates using SimpleDateFormat.
Moreover, we can change the date and time pattern (for example, dd-MM-yyyy
) according to what we need, following the specifications written in the official SimpleDateFormat documentation:
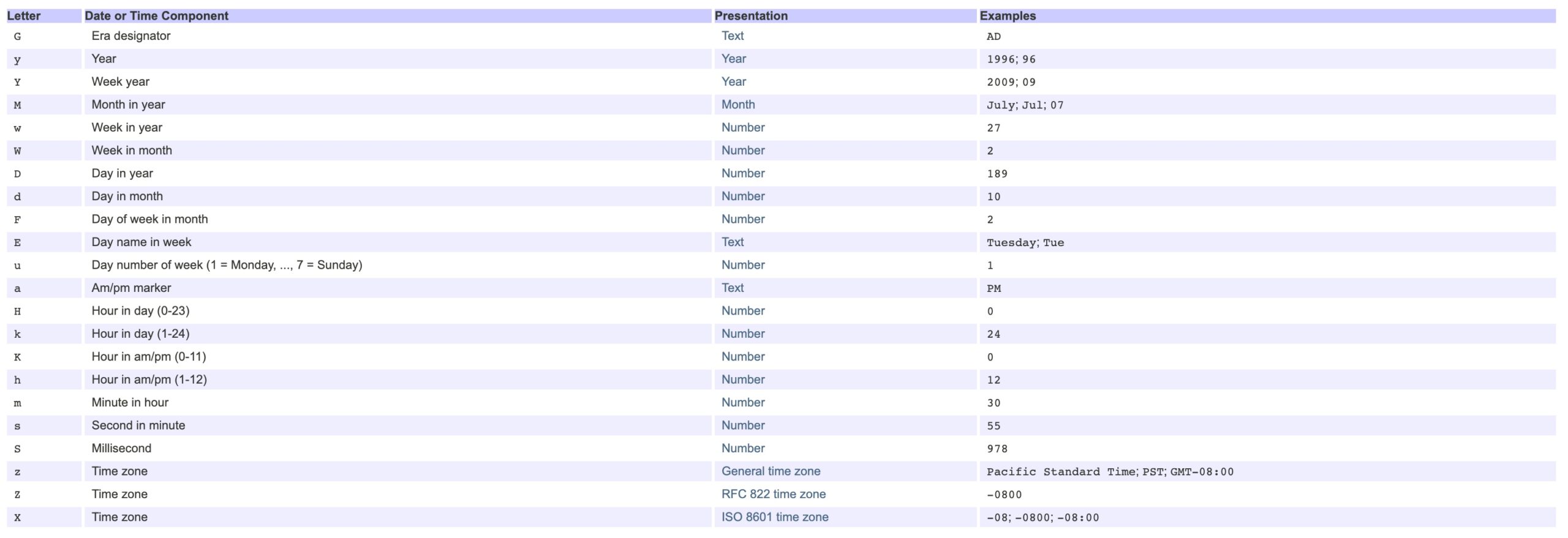
How to convert Date to String
To convert a Date to String in Java we use the method format() from the SimpleDateFormat’s class. For example, if we want to display a date as “day/month/full-year”, we use the pattern dd/MM/yyyy
like we did in the code below:
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy");
String dateStr = sdf.format(date);
Full code with a unit test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class DateToStringConversion {
public static String convertDateToString(Date date) {
SimpleDateFormat sdf = new SimpleDateFormat("dd-MM-yyyy");
return sdf.format(date);
}
@Test
@DisplayName("Convert date to string")
public void convertDateToStringTest() {
Calendar calendar = Calendar.getInstance();
calendar.set(2021, 11, 15);
Date date = calendar.getTime();
assertEquals("15-12-2021", convertDateToString(date));
}
}
How to convert a String to Date
To convert a String to Date in Java we can use the method parse()
from SimpleDateFormat.
The following code parses a String formatted using dd-MM-yyyy
pattern and returns a Date (from java.util.Date
):
SimpleDateFormat sdf = new SimpleDateFormat(datePattern);
return sdf.parse(dateStr);
Full code with a unit test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class StringToDateConversion {
public static Date stringToDateConversion(String dateStr, String datePattern) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat(datePattern);
return sdf.parse(dateStr);
}
@Test
@DisplayName("Convert String to Date")
public void stringToDateConversionTest() throws ParseException {
String dateStr = "25-02-2021";
String datePattern = "dd-MM-yyyy";
Date date = stringToDateConversion(dateStr, datePattern);
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
assertNotNull(date);
assertEquals(25, calendar.get(Calendar.DAY_OF_MONTH));
assertEquals(1, calendar.get(Calendar.MONTH));
assertEquals(2021, calendar.get(Calendar.YEAR));
}
}
How to convert a date string to a different format
To convert a date string into a different format we first have to parse the string into a Date, secondly, we format the date into a string.
The following code returns a new String with dd-MM-yyyy
pattern starting from yyyy-MM-dd
:
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat newSdf = new SimpleDateFormat("dd-MM-yyyy");
Date temp = sdf.parse(dateStr);
return newSdf.format(temp);
Full code with a unit test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class DateStringToDifferentFormat {
public static String convertDateStringToDifferentFormat(String dateStr) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat newSdf = new SimpleDateFormat("dd-MM-yyyy");
Date temp = sdf.parse(dateStr);
return newSdf.format(temp);
}
@Test
@DisplayName("Convert date string to a different format")
public void convertDateStringToDifferentFormatTest() throws ParseException {
assertEquals("15-12-1991", convertDateStringToDifferentFormat("1991-12-15"));
}
}
External Resources: